Understanding Hoisting and Closures in JavaScript
Date
18-06-2024
2 min read
Tags
Description
A comprehensive guide to understanding hoisting and closures in JavaScript.
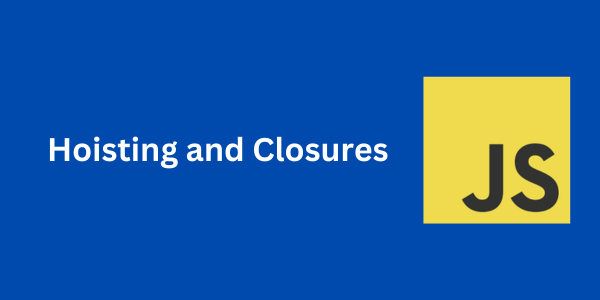
Introduction
In this blog post, we will delve into two important concepts in JavaScript: hoisting and closures. Understanding these concepts is crucial for mastering JavaScript.
What is Hoisting?
JavaScript Hoisting refers to the process whereby the interpreter appears to move the declaration of functions, variables, classes, or imports to the top of their scope, prior to execution of the code.
console.log(x); // Output: undefined
var x = 5;
console.log(x); // Output: 5
Hoisting of Functions
The below code runs without any error, despite the hoistedFunction() function being called before it's declared. This is because the JavaScript interpreter hoists the entire function declaration to the top of the current scope.
hoistedFunction(); // Output: 'This function has been hoisted.'
function hoistedFunction() {
console.log('This function has been hoisted.');
}
Understanding Closures
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log('Outer Variable: ' + outerVariable);
console.log('Inner Variable: ' + innerVariable);
};
}
const newFunction = outerFunction('outside');
newFunction('inside');
Visualizing Closures
Conclusion
In this post, we explored the concepts of hoisting and closures in JavaScript. By understanding these concepts, you can write more efficient and bug-free code. Hoisting allows variable and function declarations to be moved to the top of their scope, while closures enable functions to access variables from an outer function scope even after the outer function has closed.